Part 0:本文声明
本文只介绍基本写队列方法。
文章所有代码均为原创,转载请注明来源。
循环队列请看这里。
Part 1:啥是队列
一天,一群小可爱们在排队买奶茶。
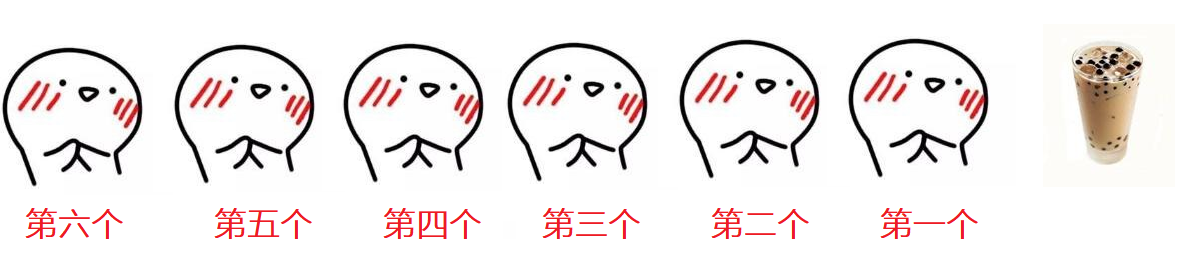
店主认识第一个小可爱,给他打了骨折(大雾),小可爱买到了奶茶,他快乐的(真的?)离开了。
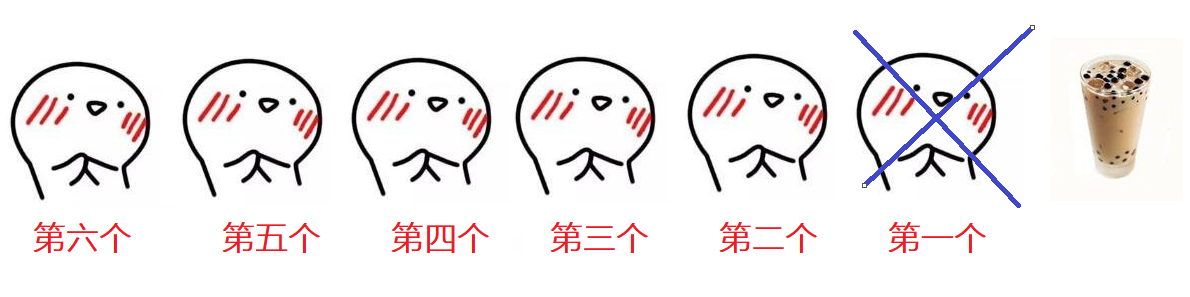
其他人往前挪一位:
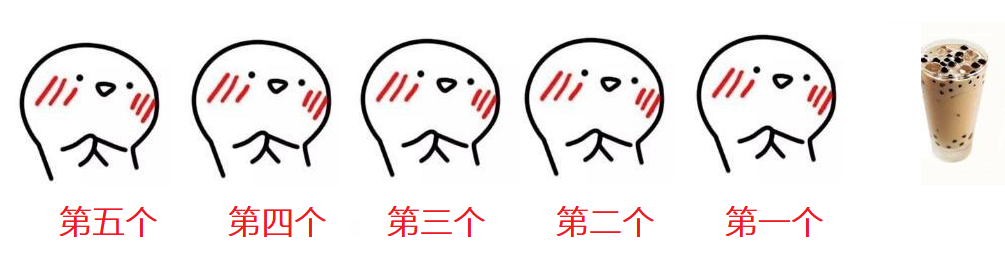
然后,目前排在第一位的小可爱买了奶茶,一蹦一跳的离开了:
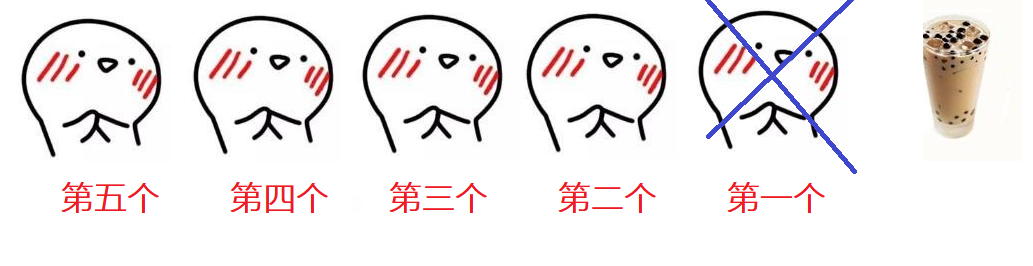
接下来,其他人往前挪一位:
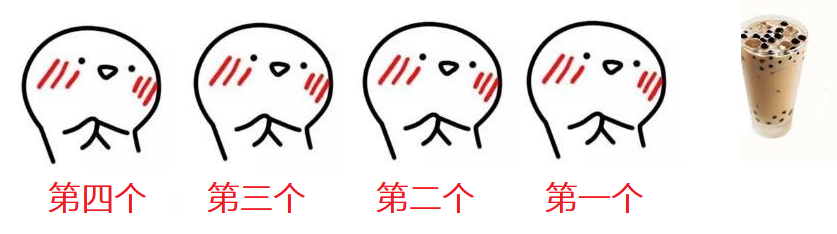
这样进行下去,最后,只剩下了一位小可爱,他买了奶茶,兴冲冲的离开了:
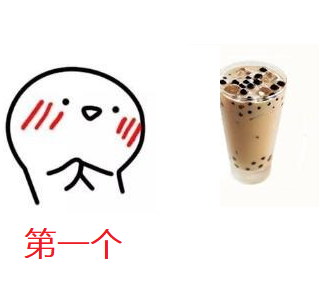
没有人啦!奶茶店卖完了奶茶,兴冲冲的关门了。
这就是队列,先进先出的数据结构。
Part 2:手写队列
如果你已经搞清楚了队列是酱紫的,我们来尝试手写一下队列。
我们简单看一下刚才排队的过程,我们可以发现,一个队列需要实现:
- 增加(有人来买奶茶)
- 弹出第一个(买完奶茶走掉)
- 求队列中元素个数(有多少个人)
- 判断队列是否为空(到底有冇得人)
- 获取队首元素(看看第一个小可爱到底是谁啊,咱给他打个骨折)
那么,我们尝试用数组和结构体来实现一下:
1 2 3 4 5 6 7 8 9 10
| struct Queue{ int que[1000]; int head = 0; int tail = 0; void push(int x){} void pop(){} bool empty(){} int num(){} int getHead(){} }
|
然后,我们来尝试写一下第一个函数push(int x)
:
1 2 3
| void push(int x){ que[tail++]=x; }
|
tail++
是个神奇的语句,不仅可以增加一个元素,并且还可以tail
指向下一个元素,增加队列的长度。
第二个:push()
,我们直接让head++
:
第三个:empty()
,直接tail-head
,因为tail
一定大于等于head
,想想为什么:
1 2 3
| bool empty(){ return tail-head; }
|
第四个num()
其实和empty()
很类似:
1 2 3
| int num(){ return tail-head; }
|
第五个也极其简单:
1 2 3
| int getHead(){ return que[head]; }
|
完整代码:
1 2 3 4 5 6 7 8 9 10
| struct Queue{ int que[1000]; int head = 0; int tail = 0; void push(int x){ que[tail++]=x; } void pop(){ head++; } bool empty(){ return tail-head; } int num(){ return tail-head; } int getHead(){ return que[head]; } }
|
我们拿去试下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| #include <iostream> using namespace std; struct Queue{ int que[1000]; int head = 0; int tail = 0; void push(int x){ que[tail++]=x; } void pop(){ head++; } bool empty(){ return tail-head; } int num(){ return tail-head; } int getHead(){ return que[head]; } }; int main(){ struct Queue queue; for(int i = 0;i < 6;i ++){ queue.push(i); } cout << queue.getHead() << endl; cout << queue.num() << endl; for(int i = 0;i < 6; i++){ queue.pop(); cout << queue.num() << ' '; cout << queue.getHead() << endl; } cout << queue.empty() << endl; }
|
程序输出:
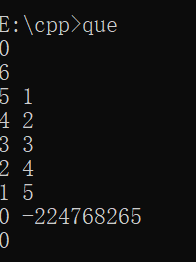
Part 3:STL里边的queue
别人也帮我们写了一个queue
,我们来看看怎么用。
1 2 3 4 5 6 7 8
| #include<queue> queue<int> q; q.push(144514); q.size(); q.front(); q.back(); q.empty(); q.pop();
|
尝试使用STL的queue写出买奶茶程序吧~